Introduction
Statistics plays a crucial role in data analysis. It helps us make sense of numbers and uncover patterns in data. Python is an excellent tool for statistical calculations due to its simplicity and powerful libraries. This article is aimed at data scientists, analysts, and anyone interested in enhancing their statistical skills using Python. We will explore Python’s statistical capabilities and how they can improve your data analysis. Learn more about statistical learning with Python.
If you’re looking to dive deeper into Python for data analysis, I highly recommend Python Crash Course by Eric Matthes. It’s a fantastic resource that balances theory and hands-on projects, making it perfect for those who want to learn quickly and effectively.
Summary and Overview
Statistics in Python encompasses a range of tools and libraries for analyzing data. Key libraries include statistics, NumPy, SciPy, and Pandas. Each library offers unique functions for statistical analysis. Understanding fundamental concepts like central tendency, variability, correlation, and inferential statistics is vital for effective analysis. Python statistics are widely used in data analysis, scientific research, and machine learning projects. These tools help extract insights, make predictions, and support decision-making across various fields.
If you want to get a solid foundation in data science concepts, grab a copy of Data Science from Scratch by Joel Grus. This book is perfect for those who want to understand the underlying principles of data science without getting lost in jargon.
Understanding Python Statistics
What is Statistics?
Statistics is the science of collecting, analyzing, interpreting, and presenting data. It provides critical insights into datasets, enabling informed decisions. Statistics is essential in various fields, including business, healthcare, and social sciences.
There are two main branches of statistics: descriptive and inferential statistics. Descriptive statistics summarize and describe the main features of a dataset. This includes measures like mean, median, and mode. On the other hand, inferential statistics allow us to make predictions or generalizations about a population based on a sample. It includes hypothesis testing and confidence intervals, which help assess the reliability of conclusions drawn from data. Understanding both branches is crucial for effective data analysis. Explore the problems with inferential statistics.
For a deeper dive into the practical applications of statistics in data science, Head First Statistics by Dawn Griffiths is a fantastic read that makes learning statistics engaging and fun!
Key Libraries for Python Statistics
When it comes to statistical analysis in Python, several libraries stand out. Each brings unique strengths to the table.
First, the statistics module is a built-in library. It provides essential statistical functions like mean, median, and mode. This module is perfect for simple calculations and small datasets.
Next up is NumPy. This library excels in numerical computing. It supports large, multi-dimensional arrays and matrices. NumPy offers high-performance mathematical functions, making it ideal for handling complex calculations. If you’re just starting, check out the NumPy Beginner’s Guide by Ivan Idris for a comprehensive introduction.
Then we have Pandas, which is fantastic for data manipulation. It provides data structures like DataFrames that allow for easy data analysis. With Pandas, you can perform operations like grouping, merging, and reshaping data effortlessly.
Lastly, SciPy builds upon NumPy. It includes advanced statistical functions and tools for scientific computing. From probability distributions to hypothesis testing, SciPy is a comprehensive resource for statisticians. For those who want a guided journey through these concepts, SciPy Cookbook by Ivan Idris is a must-read!
In summary, these libraries form the backbone of statistical analysis in Python. They enable you to perform a wide array of calculations and data manipulations efficiently. Each library complements the others, providing a robust toolkit for any data analyst or scientist.
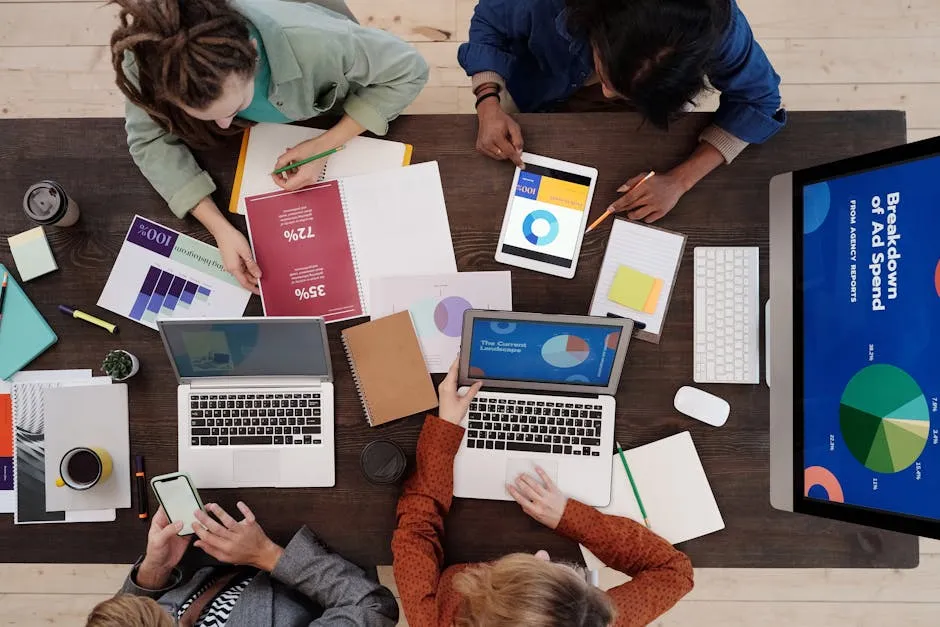
Measures of Variability
Variance
Variance measures how much individual data points differ from the mean. It’s crucial because it provides insights into data spread. A high variance indicates that data points are spread out. Conversely, a low variance suggests they are close to the mean.
To calculate variance in Python, you can use the built-in statistics module. Here’s how to do it:
import statistics
data = [10, 12, 23, 23, 16, 23, 21, 16]
variance = statistics.variance(data)
print("Variance:", variance)
This code snippet computes the sample variance for the given dataset. It’s a valuable statistic, especially when analyzing the consistency of data.

Standard Deviation
Standard deviation is the square root of variance. It indicates how much individual data points deviate from the mean in the same units as the data. A smaller standard deviation suggests that data points tend to be close to the mean, while a larger one indicates more spread out data.
To calculate standard deviation in Python, use the statistics module as follows:
import statistics
data = [10, 12, 23, 23, 16, 23, 21, 16]
std_dev = statistics.stdev(data)
print("Standard Deviation:", std_dev)
This example shows how to compute the sample standard deviation, making it easy to understand your dataset’s variability.
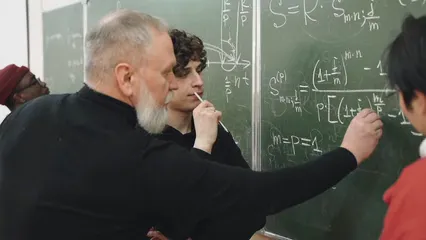
Measures of Distribution
Skewness
Skewness measures the asymmetry of a data distribution. A positive skew indicates a longer right tail, while a negative skew suggests a longer left tail. Understanding skewness helps in interpreting data distributions effectively, especially in finance and social sciences.
Here’s how to calculate skewness in Python using the scipy library:
from scipy.stats import skew
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 20]
skewness = skew(data)
print("Skewness:", skewness)
This code computes the skewness for the dataset, providing insight into its distribution. If you’re looking for a comprehensive introduction to data visualization techniques, consider Data Visualization: A Practical Introduction by Kieran Healy.
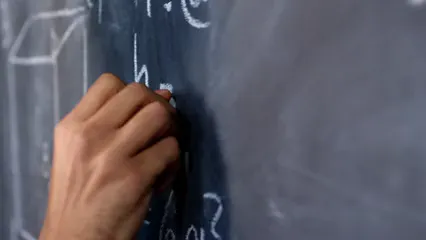
Kurtosis
Kurtosis measures the “tailedness” of a distribution. High kurtosis indicates heavy tails and outliers, while low kurtosis indicates light tails. This statistic is vital for understanding the risk of extreme values in datasets, especially in finance.
To calculate kurtosis in Python, use the following code:
from scipy.stats import kurtosis
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 20]
kurt = kurtosis(data)
print("Kurtosis:", kurt)
This example shows how to compute kurtosis, enhancing your understanding of the distribution’s characteristics. If you’re interested in expanding your statistics knowledge, I recommend Statistics for Data Science by James D. Miller.
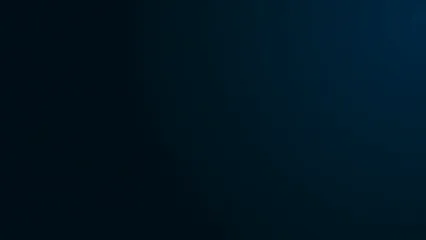
Inferential Statistics
Hypothesis Testing
Hypothesis testing is a fundamental concept in statistics. It helps us make decisions based on data. Essentially, we start with a statement, known as a hypothesis, and gather data to test its validity.
Think of it like a courtroom scenario. The null hypothesis (H0) represents the status quo, while the alternative hypothesis (H1) suggests a change. We collect evidence to see if we can reject H0 in favor of H1.
Common tests include the t-test and the chi-square test. A t-test is useful when comparing the means of two groups. For instance, if you want to know if students studying online score differently than those in class, a t-test can help. By calculating the t-statistic and comparing it to a critical value, you can determine if the difference is significant.
On the other hand, a chi-square test assesses relationships between categorical variables. For example, if you want to see if gender affects voting preferences, you would use a chi-square test. This test compares observed frequencies to expected frequencies, helping you understand if there’s a significant association.
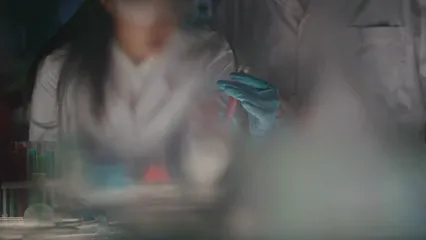
Confidence Intervals
Confidence intervals provide a range of values that likely contain the population parameter. They give us an idea of the uncertainty around our estimates. A 95% confidence interval suggests that if we were to sample repeatedly, 95% of those intervals would contain the true parameter.
Calculating confidence intervals in Python is straightforward. Using the scipy library, you can leverage its statistical capabilities. Here’s a quick example:
import numpy as np
import scipy.stats as stats
data = [20, 22, 23, 21, 25, 23, 24]
mean = np.mean(data)
sem = stats.sem(data)
ci = stats.t.interval(0.95, len(data)-1, loc=mean, scale=sem)
print("95% Confidence Interval:", ci)
This code calculates the mean and standard error of the mean, then derives the confidence interval. It’s a simple yet powerful way to understand your data.

Statistical Relationships
Correlation
Correlation measures the strength and direction of a relationship between two variables. It’s crucial for understanding how changes in one variable might influence another. A correlation coefficient ranges from -1 to +1. A value close to 1 indicates a strong positive relationship, while -1 indicates a strong negative relationship. Discover more about correlation.
In Python, you can compute correlation easily with libraries like NumPy or Pandas. For example, let’s look at Pearson and Spearman correlation coefficients.
Pearson correlation is suitable for linear relationships, while Spearman works well for non-parametric data. Here’s how to calculate both in Python:
import numpy as np
import pandas as pd
from scipy.stats import pearsonr, spearmanr
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
pearson_corr, _ = pearsonr(x, y)
spearman_corr, _ = spearmanr(x, y)
print("Pearson Correlation:", pearson_corr)
print("Spearman Correlation:", spearman_corr)
Running this code will give you both correlation coefficients, helping you interpret the relationship between the two variables. For those looking to further their understanding of statistical relationships, The Art of Data Science by Roger D. Peng and Elizabeth Matsui is a must-read!
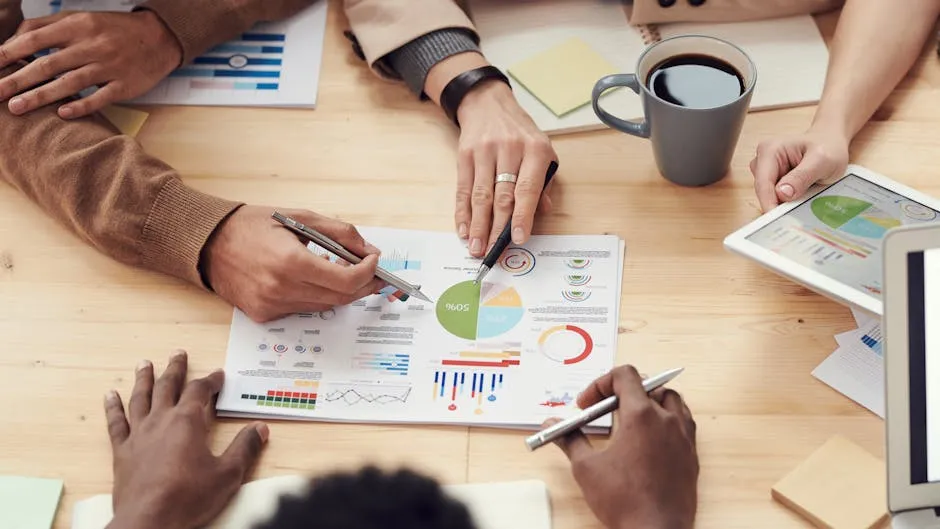
Regression Analysis
Regression analysis is a powerful statistical tool. It helps us understand the relationship between a dependent variable and one or more independent variables. By fitting a regression model, we can make predictions based on input data. Learn more about regression analysis.
For instance, simple linear regression predicts the dependent variable using a single independent variable. You can perform it easily in Python using the statsmodels library. Here’s a quick example:
import pandas as pd
import statsmodels.api as sm
data = pd.DataFrame({
'X': [1, 2, 3, 4, 5],
'Y': [2, 3, 5, 7, 11]
})
X = sm.add_constant(data['X']) # Adds a constant term to the predictor
model = sm.OLS(data['Y'], X).fit() # Fit the model
print(model.summary())
This code creates a simple linear regression model. The summary output provides insights into the relationship between X and Y, including coefficients and goodness-of-fit statistics. This analysis is essential for making informed decisions based on data.

Visualizing Data
Importance of Data Visualization
Visualizing data is essential for understanding complex statistical information. When we see data in graphical form, patterns and trends become clearer. This clarity can lead to better decision-making. Tools like Matplotlib and Seaborn are fantastic for this purpose. They allow you to create a variety of visualizations, from simple line graphs to intricate heatmaps. With these libraries, you can turn raw numbers into compelling stories. This transformation helps you share insights more effectively with others.
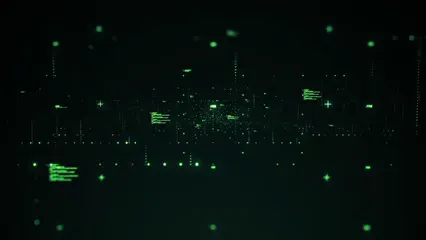
If you’re looking to master data visualization, Python Data Science Handbook by Jake VanderPlas is an excellent resource that covers not only visualization but also data manipulation and machine learning.
Creating Graphs and Charts
Creating graphs and charts in Python is straightforward, thanks to libraries like Matplotlib and Seaborn. Let’s look at some common visualizations you can easily create.
Histograms display the distribution of a dataset. To create a histogram using Matplotlib, you can use the following code:
import matplotlib.pyplot as plt
data = [1, 2, 2, 3, 3, 3, 4, 4, 5]
plt.hist(data, bins=5, alpha=0.7, color='blue')
plt.title('Histogram Example')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.show()
Box plots summarize data through their quartiles and highlight outliers. You can create a box plot like this:
import seaborn as sns
data = [1, 2, 2, 3, 3, 3, 4, 4, 5]
sns.boxplot(data=data)
plt.title('Box Plot Example')
plt.show()
Scatter plots are perfect for showing relationships between two variables. Here’s an example:
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.scatter(x, y, color='red')
plt.title('Scatter Plot Example')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
These visualizations help illustrate data insights effectively, making them invaluable tools in statistical analysis. Check out techniques for visualizing data.
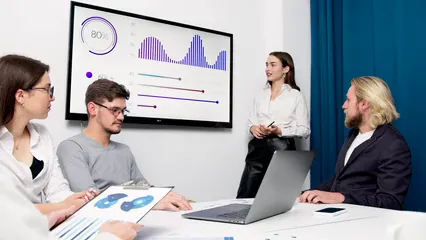
Conclusion
In summary, data visualization is a powerful method for interpreting statistical data. It allows for the quick identification of trends and patterns that might otherwise go unnoticed. Python’s libraries, like Matplotlib and Seaborn, provide the tools necessary to create impactful visualizations. I encourage you to explore these libraries further. Experiment with your datasets and see how visualizations can enhance your understanding of data analysis.
FAQs
What libraries should I use for statistical analysis in Python?
For statistical analysis in Python, consider using statistics, NumPy, Pandas, and SciPy. These libraries offer a range of functions for different statistical needs.
How do I calculate the mean and median in Python?
To calculate the mean, use statistics.mean(data). For the median, use statistics.median(data). Here’s a quick example: “`python import statistics data = [1, 2, 3, 4, 5] mean_value = statistics.mean(data) median_value = statistics.median(data) “`
What is the difference between variance and standard deviation?
Variance measures the spread of data points from the mean, while standard deviation is the square root of variance. Both provide insights into data variability, but standard deviation is in the same units as the data, making it easier to interpret.
How can I visualize statistical data in Python?
You can use libraries like Matplotlib and Seaborn for visualization. For example, use plt.hist(data) for histograms and sns.boxplot(data) for box plots. These tools help you create meaningful visual representations of your data.
What is hypothesis testing and why is it important?
Hypothesis testing is a statistical method for making inferences about populations based on sample data. It’s crucial because it allows researchers to determine if their findings are statistically significant, helping to validate assumptions or claims.
Please let us know what you think about our content by leaving a comment down below!
Thank you for reading till here 🙂
All images from Pexels