Introduction
Statistical analysis is crucial for data-driven decision-making. The Pandas library in Python makes this process straightforward. It provides powerful tools for data manipulation and analysis, making it a go-to choice for data scientists and analysts.
If you want to dive deeper into Python and understand how to leverage it for data analysis, consider picking up “Python for Data Analysis” by Wes McKinney. This book is a treasure trove of techniques and tips to master data analysis with Pandas.
Summary and Overview
This article will focus on the essential aspects of Pandas statistics. We will explore summary statistics table and various methods available in the Pandas library. Understanding these statistics is vital for gaining insights from data.
Summary statistics summarize essential characteristics of datasets. They help identify trends, patterns, and anomalies. Use cases include market research, financial analysis, and performance measurement. By leveraging Pandas, you can quickly compute these statistics and enhance your data analysis skills.
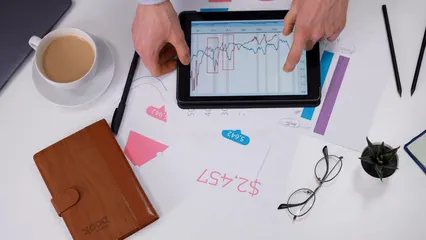
For those looking to start from scratch, a great resource is “Data Science from Scratch” by Joel Grus. This book will help you understand the foundational principles of data science using Python.
Understanding Pandas Statistics
What are Pandas Statistics?
Statistics in Pandas refers to various methods used to analyze and summarize data. This analysis is essential in data science. It helps in interpreting data and making predictions.
Pandas offers two main types of statistics: descriptive and inferential. Descriptive statistics summarize the central tendency and distribution of data. They include measures like mean, median, and standard deviation. In contrast, inferential statistics allow us to make predictions or generalizations about a population based on a sample. For more insights on this, you can check the problem with inferential statistics.
These statistical tools are invaluable for data scientists. They enable efficient data analysis and informed decision-making. By mastering these techniques, you can extract meaningful insights from vast datasets. Plus, for a comprehensive guide on leveraging Pandas, consider the “Pandas Cookbook” by Theodore Petrou. It’s packed with practical recipes for data manipulation!
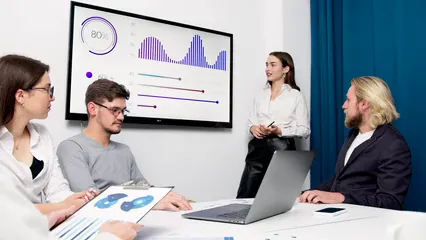
The Importance of Summary Statistics
Summary statistics play a vital role in data analysis. They provide a concise overview of key characteristics in your dataset. By summarizing data, you can quickly identify trends, patterns, and outliers. This understanding is crucial when making informed decisions.
When you analyze a dataset, summary statistics help you grasp data distributions. For example, knowing the mean and standard deviation offers insight into central tendencies and variability. If you’re examining sales data, these statistics can reveal how consistent your revenue is over time. For a deeper understanding of what mean means in statistics, see what does mean identically distributed in statistics.
Consider a company analyzing its employee performance metrics. By using summary statistics, management can pinpoint the average performance score and the distribution of scores. This helps in identifying high and low performers, guiding recruitment and training decisions. Overall, summary statistics enhance your ability to interpret data effectively, leading to better outcomes in various fields. If you’re looking for a solid foundation on the statistical principles behind data science, check out “Data Science for Business” by Foster Provost and Tom Fawcett. It’s the perfect blend of theory and application!

Standard Deviation and Variance
Standard deviation and variance are essential statistics. They measure how data points differ from the mean. Variance shows the average of squared differences. The standard deviation is simply the square root of variance.
In Pandas, you can calculate these values easily. For variance, use the var()
function. For standard deviation, the std()
function works perfectly. Here’s how you can do it:
import pandas as pd
data = {'values': [10, 12, 23, 23, 16, 23, 21]}
df = pd.DataFrame(data)
variance = df['values'].var()
std_dev = df['values'].std()
print("Variance:", variance)
print("Standard Deviation:", std_dev)
Understanding these metrics is crucial for data analysis. They help in assessing the spread of data. For example, a low standard deviation indicates that values are close to the mean. In contrast, a high standard deviation suggests that values are widely spread out. This insight helps in making data-driven decisions. For a deeper dive into statistics, you might also consider “Practical Statistics for Data Scientists” by Peter Bruce and Andrew Bruce, which offers a hands-on approach.
Correlation and Covariance
Correlation and covariance help analyze relationships between variables. Correlation indicates how strongly two variables are related. Covariance shows the direction of their relationship. For a better understanding of correlation, refer to correlation.
In Pandas, you can compute these matrices easily with the corr()
and cov()
functions. Here’s an example of how to calculate them:
import pandas as pd
data = {
'A': [1, 2, 3, 4],
'B': [5, 6, 7, 8],
'C': [9, 10, 11, 12]
}
df = pd.DataFrame(data)
correlation_matrix = df.corr()
covariance_matrix = df.cov()
print("Correlation Matrix:\n", correlation_matrix)
print("Covariance Matrix:\n", covariance_matrix)
These metrics are vital for understanding data dynamics. A high correlation value indicates a strong relationship, while a low value suggests otherwise. These insights are particularly useful in predictive modeling and risk assessment. To further enhance your predictive modeling skills, consider “Python Machine Learning” by Sebastian Raschka. It’s a fantastic resource!
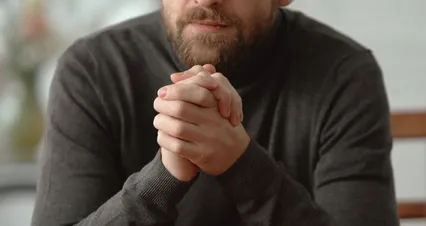
Advanced Statistical Operations
Grouping Data and Aggregating Statistics
Grouping data in Pandas is powerful for analysis. You can summarize data based on categories. This is done using the groupby()
function. It allows you to split the data into groups and perform aggregations.
For example, consider a dataset of sales data. You can group by product categories and calculate total sales. Here’s how:
import pandas as pd
data = {
'Category': ['A', 'B', 'A', 'B', 'A', 'B'],
'Sales': [100, 200, 150, 250, 300, 350]
}
df = pd.DataFrame(data)
grouped = df.groupby('Category')['Sales'].agg(['sum', 'mean', 'count'])
print(grouped)
In this example, the groupby()
function organizes the data by category. The agg()
function computes total sales, average sales, and counts of entries for each category.
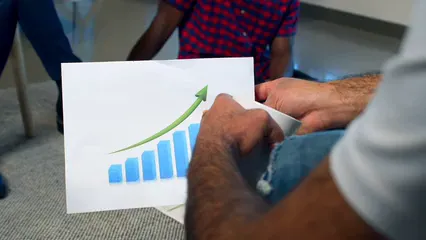
Grouping data provides valuable insights. It helps identify trends and performance across different segments. By understanding these metrics, businesses can tailor strategies to improve performance. This approach is widely applicable in marketing, finance, and operations. If you’re eager to learn more about data visualization techniques, check out “Data Visualization: A Practical Introduction” by Kieran Healy. It’s filled with useful examples!
Custom Percentiles and Quantiles
Calculating custom percentiles in Pandas is straightforward. You can use the quantile()
method from a DataFrame or Series. Percentiles indicate the relative standing of a value within a dataset. For example, the 25th percentile means that 25% of the data points are below this value.
Quantiles divide the data into equal-sized intervals. The key difference is that percentiles are specific quantiles. For instance, the median (50th percentile) is also referred to as the second quartile.
Here’s how to calculate custom percentiles in Pandas:
import pandas as pd
data = {'values': [10, 20, 30, 40, 50, 60]}
df = pd.DataFrame(data)
# Calculate the 20th and 80th percentiles
percentiles = df['values'].quantile([0.2, 0.8]).to_dict()
print("Custom Percentiles:", percentiles)
This code snippet demonstrates how to compute specific percentiles. Adjust the quantiles in the list to calculate other values as needed. And if you’re interested in a broader understanding of data mining, consider “Data Mining: Concepts and Techniques” by Jiawei Han and Micheline Kamber.
Visualizing Summary Statistics
Visualization Techniques for Statistical Data
Visualizing summary statistics is essential for understanding your dataset. Charts and graphs provide insights that raw numbers cannot. They help you quickly identify trends and patterns in your data.
Libraries like Matplotlib and Seaborn are popular for creating visualizations. Matplotlib is versatile and supports various chart types. Seaborn enhances your plots with attractive default styles and color palettes. If you’re looking to get started with these libraries, check out “Matplotlib for Python Developers” by Sandro Tosi. It’s a fantastic guide!
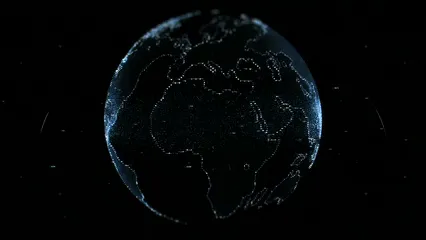
Here are examples of visualizing statistical outputs:
1. Histogram: Displays the distribution of a single variable.
import matplotlib.pyplot as plt
plt.hist(df['values'], bins=5, alpha=0.7, color='blue')
plt.title('Histogram of Values')
plt.xlabel('Values')
plt.ylabel('Frequency')
plt.show()
2. Box Plot: Shows the summary statistics of a dataset, highlighting the median, quartiles, and potential outliers.
import seaborn as sns
sns.boxplot(data=df['values'])
plt.title('Box Plot of Values')
plt.show()
These visualizations make it easier to interpret your data. They provide a clear overview of data distributions and potential anomalies. Engaging with visual data representations can enhance your analytical skills and decision-making process. For a great introduction to statistical data visualization, consider “Seaborn: Statistical Data Visualization”. It’s an essential read!

Conclusion
Understanding and calculating statistics using Pandas is essential for effective data analysis. With its powerful functionality, Pandas simplifies the process of deriving insights from complex datasets. By mastering summary statistics, you can quickly identify trends, patterns, and anomalies in your data. This knowledge aids in making informed, data-driven decisions across various domains, from finance to healthcare. I encourage you to leverage these tools and integrate them into your analytical toolbox. Embrace the power of Pandas to enhance your data analysis skills and drive your projects forward. If you’re keen on diving deeper into data science, consider “The Art of Data Science” by Roger D. Peng and Elizabeth Matsui. It’s a fantastic overview!
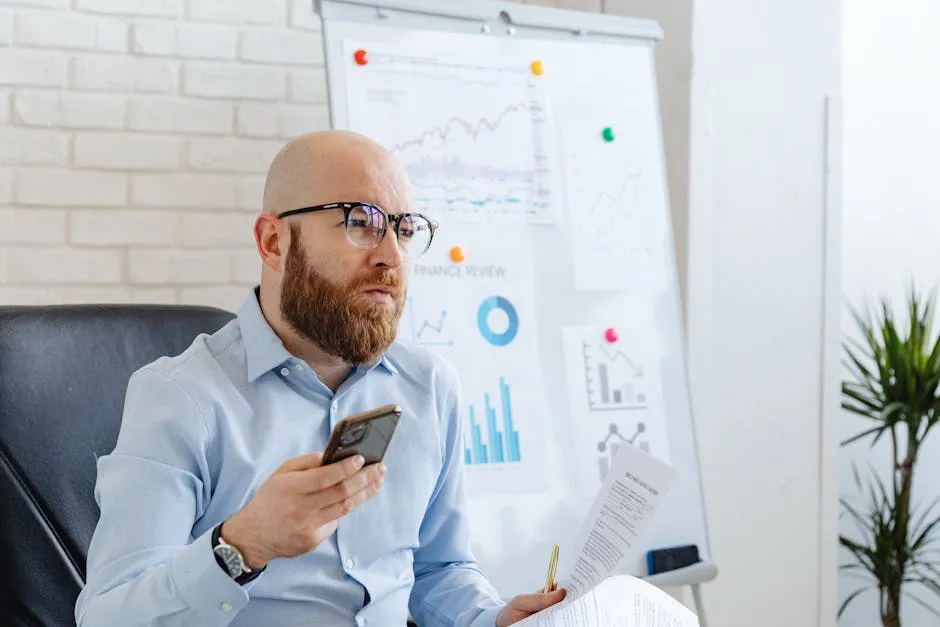
FAQs
What is the `describe()` function in Pandas?
The `describe()` function generates descriptive statistics for DataFrames. It provides insights such as count, mean, standard deviation, minimum, maximum, and specified percentiles. This method is useful for quickly summarizing numerical data.
How do you calculate median in Pandas?
To compute the median in Pandas, use the `median()` function. For example, `df[‘column_name’].median()` yields the median value of the specified column. The median is significant as it represents the middle point of a dataset, minimizing the influence of outliers. For further details, see statistics poland median salary 2024.
Can I calculate statistics for specific columns in a DataFrame?
Yes, you can calculate statistics for specific columns by selecting them first. For instance, use `df[[‘column1’, ‘column2’]].describe()` to obtain summary statistics only for the chosen columns.
What are the differences between `mean()` and `median()`?
The `mean()` function calculates the average of values, while `median()` finds the middle value when data is sorted. Use mean for normally distributed data and median for skewed distributions to avoid distortion from outliers.
How do I handle missing values when calculating statistics in Pandas?
When dealing with missing values, you can use methods like `dropna()` to exclude them or `fillna()` to replace them with a specific value. These strategies ensure accurate calculations without the influence of NaN values.
What visualization techniques are best for representing summary statistics?
Visualizations such as histograms and box plots effectively represent summary statistics. Histograms show data distribution, while box plots highlight medians, quartiles, and potential outliers. Both can enhance understanding of your data.
How can I export summary statistics to a CSV file?
To export summary statistics to a CSV file, you can use the `to_csv()` method. For example, `df.describe().to_csv(‘summary_statistics.csv’)` will save the summary statistics to a CSV file named “summary_statistics.csv”.
Please let us know what you think about our content by leaving a comment down below!
Thank you for reading till here 🙂
All images from Pexels