Introduction
Have you ever wondered how data standards shape educational assessment? The Ed-Fi data standard plays a crucial role in this area. Its assessment domain captures vital information about student evaluations. Inserting data accurately into this domain is key for effective education analytics. This article provides sample SQL commands to help you insert data efficiently.
To dive deeper into the Ed-Fi data standard, check out Ed-Fi Data Standard: A Practical Guide. This book is like having a GPS for navigating the complex world of educational data standards—minus the annoying voice telling you to make a U-turn!
Summary and Overview
The Ed-Fi assessment domain encompasses various elements related to student evaluations. It includes assessments, scores, and results. This domain integrates seamlessly into the broader Ed-Fi framework, promoting data interoperability. Educators and developers must input assessment data to ensure accurate reporting and analysis. Below, we’ll introduce SQL commands that streamline this process. Using sample SQL commands can significantly enhance data integration and management, making your work easier and more efficient.
For those looking to amplify their SQL skills, consider SQL for Data Analysis: Advanced Techniques for SQL Queries. This book is perfect for those who want to take their SQL game to the next level and impress their colleagues with their newfound data wizardry.
Understanding the Ed-Fi Assessment Domain
What is the Ed-Fi Assessment Domain?
The Ed-Fi assessment domain is essential for managing educational assessments. It serves as a structured environment for storing assessment data, including tests and evaluations. Key components of this domain include assessment types, results, and student performance metrics. Ensuring data accuracy in this domain is vital. Accurate data helps educators make informed decisions based on reliable insights. When data integrity is maintained, it enhances the overall educational experience for students.
If you’re new to SQL, you might find Learning SQL: Master SQL Fundamentals a great read. It’s a friendly introduction to SQL that will have you querying like a pro in no time. No more Googling “how to write a SELECT statement” at 2 AM!
Structure of the Assessment Domain
Understanding the structure of the assessment domain is crucial for effective data management. This domain includes several key data entities, such as assessments and their results. Each entity interacts with others to form a cohesive framework. For example, an assessment entity may relate to multiple results based on different student performances. Recognizing these relationships is important before inserting data. Comprehending the data structure ensures you can input information accurately and efficiently, leading to better educational outcomes.
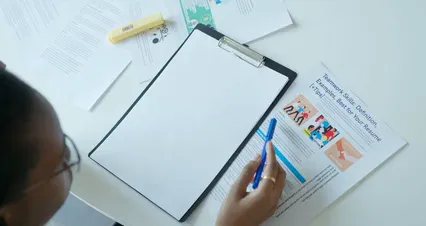
Preparing Your SQL Environment
Setting Up Your Database
Before you can insert data into the Ed-Fi assessment domain, you need a properly configured database. Start by selecting a SQL environment. MySQL and PostgreSQL are solid choices due to their popularity and support.
First, create your database. Use the following command:
CREATE DATABASE edfi_assessment;
Next, switch to your new database:
USE edfi_assessment;
Now, create the necessary tables. Structure your environment to handle data efficiently. Proper configuration makes data management smoother. Check your server settings and ensure they align with your project needs. This setup is crucial for effective data handling.
If you’re looking for a great resource on PostgreSQL, check out PostgreSQL: Up and Running. This book will get you up to speed with PostgreSQL faster than you can say “SELECT * FROM my_table.”
Sample Table Structures for Assessment Data
To work with assessment data, you’ll need specific tables. Here’s a basic outline of the tables you should consider:
- Assessments: Store details about each assessment.
- Results: Record student results tied to assessments.
- Students: Keep track of student identifiers.
You can create these tables with the following SQL commands:
CREATE TABLE Assessments (
AssessmentID INT PRIMARY KEY,
AssessmentName VARCHAR(255),
AssessmentDate DATE
);
CREATE TABLE Results (
ResultID INT PRIMARY KEY,
AssessmentID INT,
StudentID INT,
Score DECIMAL(5,2),
FOREIGN KEY (AssessmentID) REFERENCES Assessments(AssessmentID)
);
CREATE TABLE Students (
StudentID INT PRIMARY KEY,
StudentName VARCHAR(255)
);
When designing your tables, prioritize scalability. Keep your data types efficient and ensure relationships are clear. This approach helps future-proof your database and enhances performance as your data grows.

Sample SQL Commands for Data Insertion
Basic SQL Insert Command
Inserting data is straightforward with SQL. Here’s a simple command to add an assessment record:
INSERT INTO Assessments (AssessmentID, AssessmentName, AssessmentDate)
VALUES (1, 'Math Test', '2023-10-01');
Each field plays a role. The AssessmentID identifies the record, while AssessmentName describes it. The AssessmentDate indicates when the assessment took place. This structure is essential for tracking and reporting.
For those who want to dive deeper into SQL performance, SQL Performance Explained is a great read. It’s like having a personal trainer for your SQL queries—minus the sweat!
Inserting Multiple Records
Batch inserts can save time and improve performance. Here’s how to insert multiple records at once:
INSERT INTO Assessments (AssessmentID, AssessmentName, AssessmentDate)
VALUES
(2, 'Science Test', '2023-10-02'),
(3, 'History Test', '2023-10-03');
Using batch inserts reduces the number of calls to the database. This efficiency is crucial for larger datasets. It helps maintain performance during data uploads, ensuring your application runs smoothly even under heavy loads.

Using Transactions for Data Integrity
Transactions are vital for maintaining data integrity in database operations. They ensure that a series of SQL commands are executed as a single unit. If one command fails, the entire transaction can be rolled back, preventing partial updates. This guarantees that your database remains consistent.
For example, when inserting assessment data, you might want to insert a new assessment and its corresponding results in one transaction. Here’s how you can do it:
START TRANSACTION;
INSERT INTO Assessments (AssessmentID, AssessmentName, AssessmentDate)
VALUES (1, 'Math Test', '2023-10-01');
INSERT INTO Results (ResultID, AssessmentID, StudentID, Score)
VALUES (1, 1, 101, 85.0);
COMMIT;
In this scenario, if either insert fails, you can call ROLLBACK to revert all changes. This ensures that your assessment data remains complete and accurate.
Handling Errors and Rollbacks
Errors can occur during data insertion, such as constraint violations or data type mismatches. These errors can disrupt your data integrity. Handling these errors effectively is essential for smooth operation.
For instance, consider the following example:
BEGIN;
INSERT INTO Results (ResultID, AssessmentID, StudentID, Score)
VALUES (1, 1, 101, 85.0);
IF @@ERROR <> 0
BEGIN
ROLLBACK;
PRINT 'Insertion failed, changes have been rolled back.';
END
ELSE
BEGIN
COMMIT;
PRINT 'Insertion successful.';
END
In this SQL code, we use BEGIN and IF @@ERROR to check for errors after the insert. If an error is detected, we roll back the transaction, ensuring that no invalid data is saved.

Best Practices for Data Insertion
Data Validation Techniques
Validating data before insertion is crucial. It prevents issues such as incorrect data types or missing values. By checking data upfront, you can enhance the reliability of your database.
For example, you can use SQL constraints for validation:
CREATE TABLE Results (
ResultID INT PRIMARY KEY,
AssessmentID INT NOT NULL,
StudentID INT NOT NULL,
Score DECIMAL(5,2) CHECK (Score BETWEEN 0 AND 100)
);
In this command, we ensure that the Score is always between 0 and 100. This type of validation helps maintain high data quality.

Maintaining Data Integrity
Data integrity is essential for accurate information storage. Primary keys, foreign keys, and constraints play a significant role. They help enforce relationships between tables and ensure data correctness.
Here’s how you can define these rules:
CREATE TABLE Assessments (
AssessmentID INT PRIMARY KEY,
AssessmentName VARCHAR(255) NOT NULL,
AssessmentDate DATE NOT NULL
);
CREATE TABLE Results (
ResultID INT PRIMARY KEY,
AssessmentID INT,
StudentID INT,
Score DECIMAL(5,2),
FOREIGN KEY (AssessmentID) REFERENCES Assessments(AssessmentID)
);
With these commands, we establish relationships between Assessments and Results. These constraints ensure that every result corresponds to a valid assessment, maintaining data integrity across the board.

Optimizing SQL Performance
When working with SQL, indexing can greatly enhance data retrieval speed. Indexes are like roadmaps for your database. They allow the database engine to find data without scanning every row. This can make a big difference in performance, especially with large datasets.
To optimize your SQL queries, consider the following tips:
- Use SELECT only for needed columns: Instead of using SELECT *, specify the columns you need. This reduces the amount of data processed and sent over the network.
- Limit the number of JOINs: While JOINs are necessary, excessive use can slow down queries. Keep your JOINs to a minimum and ensure that they are on indexed columns.
- Utilize WHERE clauses: Filtering data early in the query allows SQL to work with less data. This can lead to faster execution times.
- Analyze your queries: Use SQL execution plans to identify slow queries. This insight helps you understand where optimizations are needed.
- Batch inserts: When inserting multiple rows, use a single INSERT statement. This reduces overhead and speeds up the process.
By implementing these strategies, you’ll notice improved performance in your SQL operations. And speaking of performance, if you want to keep your workspace neat while working on your SQL projects, consider the Logitech MX Master 3 Advanced Wireless Mouse. It’s the best thing since sliced bread for your productivity!

Conclusion
Inserting data correctly into the Ed-Fi assessment domain using SQL is crucial for maintaining data integrity and accuracy. Following best practices, like optimizing your SQL commands and using sample SQL effectively, can streamline data management. Embrace these techniques to enhance your work within the Ed-Fi framework.
FAQs
What is the Ed-Fi assessment domain used for?
The Ed-Fi assessment domain tracks educational evaluations. It allows educators to manage and analyze student performance data effectively.
How do I know if my SQL commands are correct?
To validate SQL commands, you can use tools like SQL linters or run them in a safe testing environment. Additionally, check for syntax errors and ensure proper data types before execution.
Can I modify the sample SQL commands provided?
Absolutely! The sample SQL commands are just a starting point. You can adjust them to fit your specific needs. For instance, you might need to modify field names to match your database schema. Feel free to add more records or change data types as required. The flexibility of SQL allows you to tailor these commands for your unique educational context.
What should I do if I encounter errors during data insertion?
Errors during data insertion can be frustrating but manageable. Start by checking your SQL syntax for any mistakes. Ensure that all data types match your table definitions. If you see a constraint violation, verify that you’re not breaking any foreign key rules. Use error messages to guide your troubleshooting. Additionally, resources like SQL documentation and community forums can provide insights and solutions.
Is there a way to automate data insertion into the Ed-Fi system?
Yes, automation is possible and can save you a lot of time. You can use scripts or tools like Python or PowerShell to automate the data insertion process. Tools such as Data Loader or API integrations can also help streamline this task. By automating, you can focus more on analysis rather than manual data entry.
How does data integrity impact educational assessments?
Data integrity is crucial for reliable educational assessments. It ensures that the data collected is accurate and trustworthy. When data integrity is compromised, it can lead to incorrect conclusions about student performance. This, in turn, can affect teaching strategies and educational policies. Maintaining high data integrity fosters confidence in assessment results and supports better decision-making.
Are there any resources for learning more about Ed-Fi SQL commands?
Certainly! The Ed-Fi Alliance offers extensive documentation on SQL commands and their application within the Ed-Fi framework. Online tutorials and forums can also provide practical insights. Websites like GitHub often host community-driven resources and examples. Don’t hesitate to explore these materials to deepen your understanding of Ed-Fi SQL commands.
Please let us know what you think about our content by leaving a comment down below!
Thank you for reading till here 🙂 And if you’re looking for a way to relax after all this SQL talk, consider treating yourself to a Fire HD 10 Tablet. It’s perfect for binge-watching your favorite shows or reading that next great book!
All images from Pexels