Introduction
Statistical analysis plays a crucial role in data science. It helps us extract meaningful insights from vast amounts of data. Python stands out as a powerful tool for this purpose. Its simplicity and rich ecosystem of libraries make it ideal for statistical tasks. This article aims to provide a thorough overview of Python’s capabilities in statistical analysis, covering key concepts and tools.
If you’re looking for a great starting point, consider picking up Python Crash Course: A Hands-On, Project-Based Introduction to Programming. It’s perfect for beginners who want to dive into Python and start analyzing data like a pro!
Summary and Overview
Statistical analysis involves techniques for understanding data. It helps in summarizing, interpreting, and drawing conclusions from datasets. Python libraries, like NumPy and Pandas, facilitate smooth statistical analysis. We’ll discuss major topics like descriptive statistics, inferential statistics, and more. Python’s popularity continues to rise in the data science community, making it essential for practitioners.
If you’re keen on data science, check out Data Science from Scratch: First Principles with Python. It breaks down the fundamentals of data science in a bite-sized manner, making it a must-read!
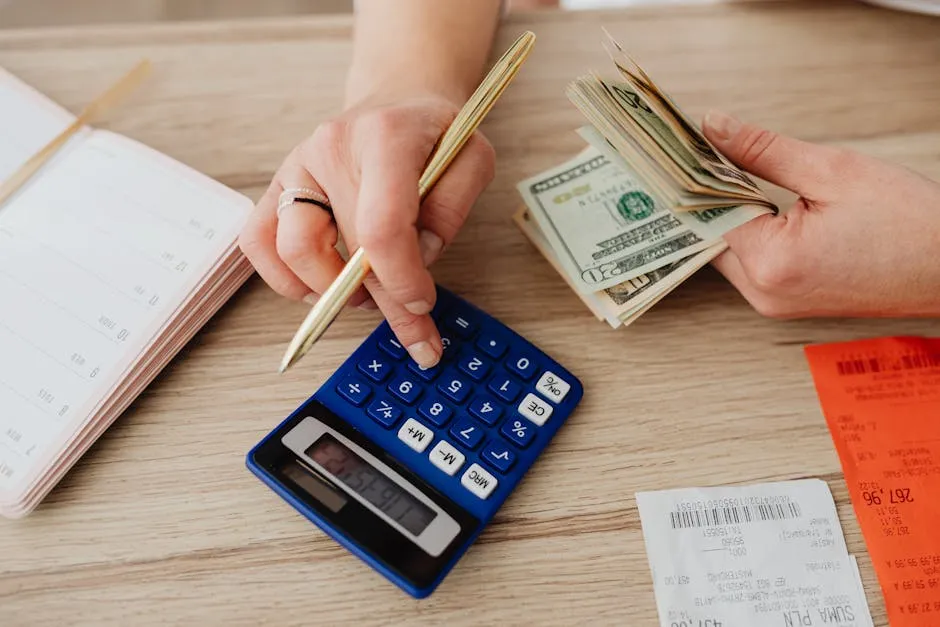
Types of Statistical Analysis in Python
Descriptive Statistics
Descriptive statistics summarize data features effectively. It includes key metrics such as mean, median, mode, variance, and standard deviation. These metrics provide insights into the central tendency and variability of data.
Python libraries like Pandas and NumPy are essential for calculating these statistics. For example, you can compute the mean and standard deviation easily using these libraries. Here’s a simple code snippet to illustrate this:
import pandas as pd
data = [10, 20, 30, 40, 50]
df = pd.Series(data)
mean_value = df.mean()
std_dev = df.std()
print("Mean:", mean_value)
print("Standard Deviation:", std_dev)
This code calculates the mean and standard deviation of a dataset. By using these tools, you can quickly summarize your data and gain valuable insights. Descriptive statistics is often the first step in understanding any dataset before proceeding to deeper analyses.
For a deeper dive into statistical concepts, grab Practical Statistics for Data Scientists: 50 Essential Concepts. This book breaks down the essential statistical concepts you need to know in the field.
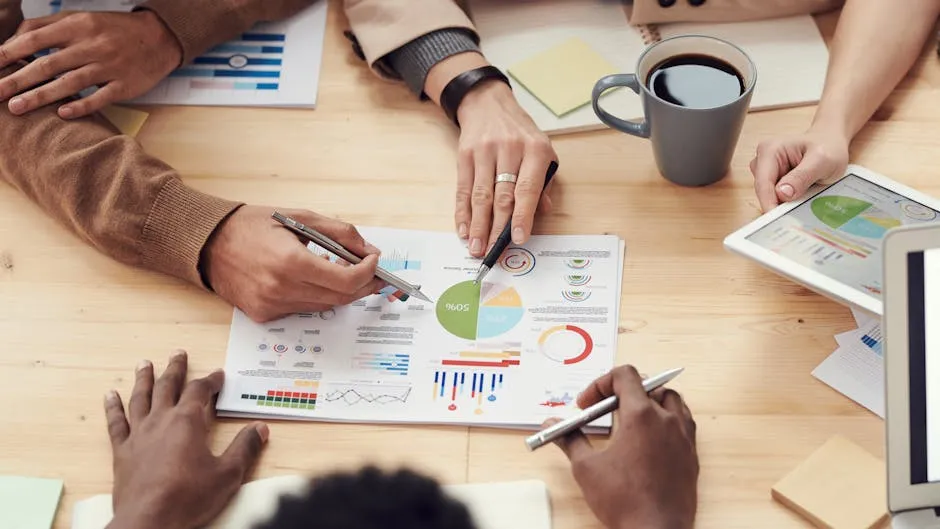
Inferential Statistics
Inferential statistics allows us to make predictions about a population based on a sample. It plays a crucial role in hypothesis testing. This process helps us determine whether a specific claim about a dataset holds true.
Common tests in inferential statistics include t-tests, ANOVA, and chi-square tests. A t-test compares the means of two groups to see if they are significantly different. ANOVA extends this idea to compare means across three or more groups. Chi-square tests assess the relationship between categorical variables.
In Python, libraries like SciPy and StatsModels are valuable for conducting these tests. For instance, you can use SciPy’s ttest_ind
function for a t-test. Here’s a simple example:
from scipy import stats
group1 = [1, 2, 3, 4, 5]
group2 = [2, 3, 4, 5, 6]
t_stat, p_value = stats.ttest_ind(group1, group2)
print("T-statistic:", t_stat)
print("P-value:", p_value)
For ANOVA, you might use:
import statsmodels.api as sm
from statsmodels.formula.api import ols
data = {'values': [1, 2, 3, 4, 5, 6], 'group': ['A', 'A', 'B', 'B', 'C', 'C']}
model = ols('values ~ group', data=data).fit()
anova_table = sm.stats.anova_lm(model, typ=2)
print(anova_table)
These examples illustrate how easily you can apply inferential statistics using Python. To understand more about the problems related to inferential statistics, you can read this article:
Learn about the challenges in inferential statistics in this guide: the problem with inferential statistics.
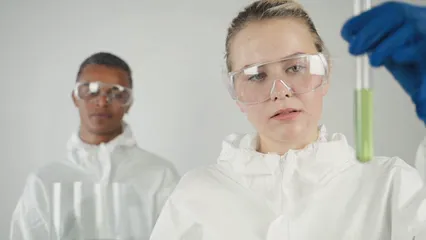
Correlation and Regression Analysis
Correlation measures the strength and direction of a relationship between two variables. It helps understand how one variable may change in relation to another. A correlation coefficient ranges from -1 to 1, indicating perfect negative to perfect positive correlation.
Regression analysis builds on this concept. It allows you to model the relationship between a dependent variable and one or more independent variables. Types of regression include linear and logistic regression. Linear regression predicts a continuous outcome, while logistic regression is used for binary outcomes.
To perform correlation and regression in Python, you can use StatsModels and Scikit-learn. Here’s an example of calculating correlation:
import numpy as np
import pandas as pd
data = pd.DataFrame({
'x': [1, 2, 3, 4, 5],
'y': [2, 3, 5, 7, 11]
})
correlation = data['x'].corr(data['y'])
print("Correlation coefficient:", correlation)
For linear regression, you might write:
from sklearn.linear_model import LinearRegression
X = data[['x']]
y = data['y']
model = LinearRegression().fit(X, y)
print("Coefficient:", model.coef_)
print("Intercept:", model.intercept_)
You can visualize the results with a scatter plot and a regression line using Matplotlib:
import matplotlib.pyplot as plt
plt.scatter(data['x'], data['y'], color='blue')
plt.plot(data['x'], model.predict(X), color='red')
plt.xlabel('X')
plt.ylabel('Y')
plt.title('Linear Regression')
plt.show()
These techniques enable you to uncover relationships in your data effectively. For a deeper understanding of regression analysis, refer to this comprehensive guide:
Explore this detailed guide on regression analysis: regression analysis.
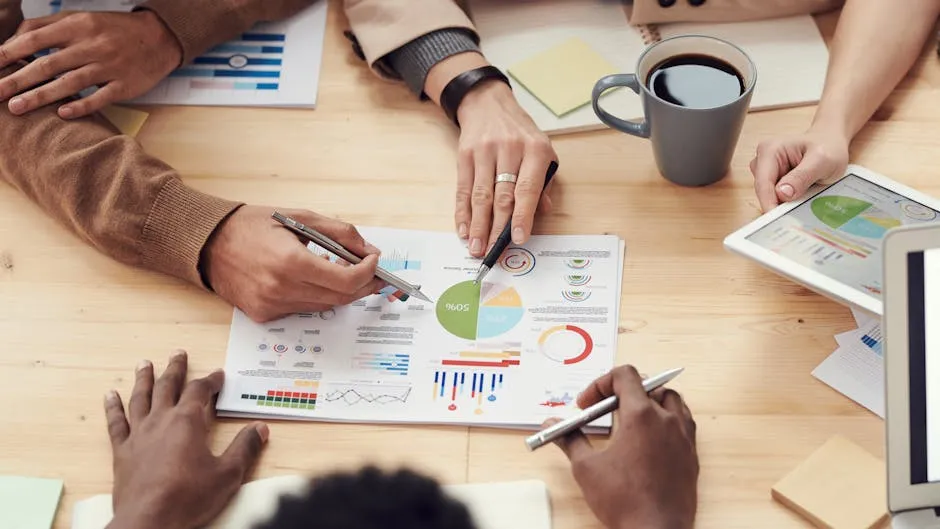
Multivariate Statistics
Multivariate statistics analyzes data with multiple variables. This method is vital for understanding complex datasets. It helps reveal relationships and patterns that single-variable analysis might miss.
Principal Component Analysis (PCA) is a popular technique used in multivariate statistics. PCA reduces data dimensionality while preserving variance. It simplifies data visualization and helps identify key trends. Another method is cluster analysis. This technique groups similar data points together, revealing underlying structures.
In Python, libraries like Scikit-learn and StatsModels are essential for multivariate analysis. Scikit-learn provides functions for PCA and clustering. Here’s an example of PCA in action:
from sklearn.decomposition import PCA
from sklearn.datasets import load_iris
import pandas as pd
# Load dataset
data = load_iris()
X = data.data
# Perform PCA
pca = PCA(n_components=2)
X_reduced = pca.fit_transform(X)
df = pd.DataFrame(X_reduced, columns=['PC1', 'PC2'])
print(df.head())
This code snippet demonstrates how to reduce the dimensions of the Iris dataset using PCA. It creates a new dataset with two principal components, making further analysis simpler.
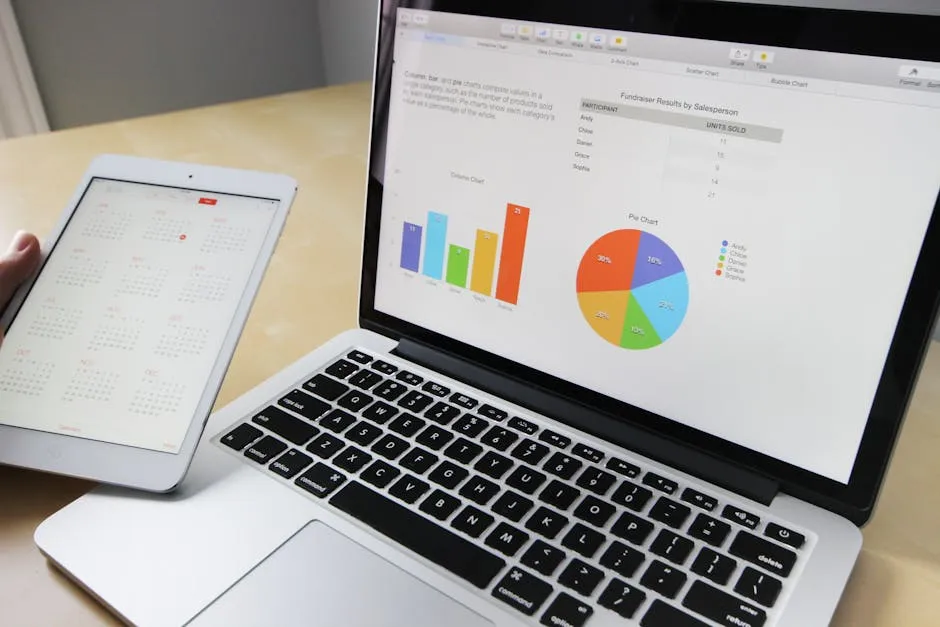
Statistical Modeling
Statistical modeling uses mathematical frameworks to describe relationships in data. It enables predictions and hypothesis testing, making it a powerful tool in data analysis.
Common techniques include linear regression and logistic regression. Linear regression predicts a continuous outcome, while logistic regression is used for binary outcomes. These models help quantify relationships between variables.
Python libraries like StatsModels and Scikit-learn facilitate statistical modeling. StatsModels focuses on statistical tests and provides detailed output. Here’s a step-by-step example using linear regression in Python:
import pandas as pd
import statsmodels.api as sm
# Load dataset
data = pd.read_csv('data.csv')
X = data[['feature1', 'feature2']]
y = data['target']
# Add constant for intercept
X = sm.add_constant(X)
# Fit model
model = sm.OLS(y, X).fit()
print(model.summary())
In this example, we load a dataset, specify features and target, and fit a linear regression model. The summary provides insights into the relationships between variables. For more on linear regression, refer to this guide:
Learn about linear regression in detail: linear regression.
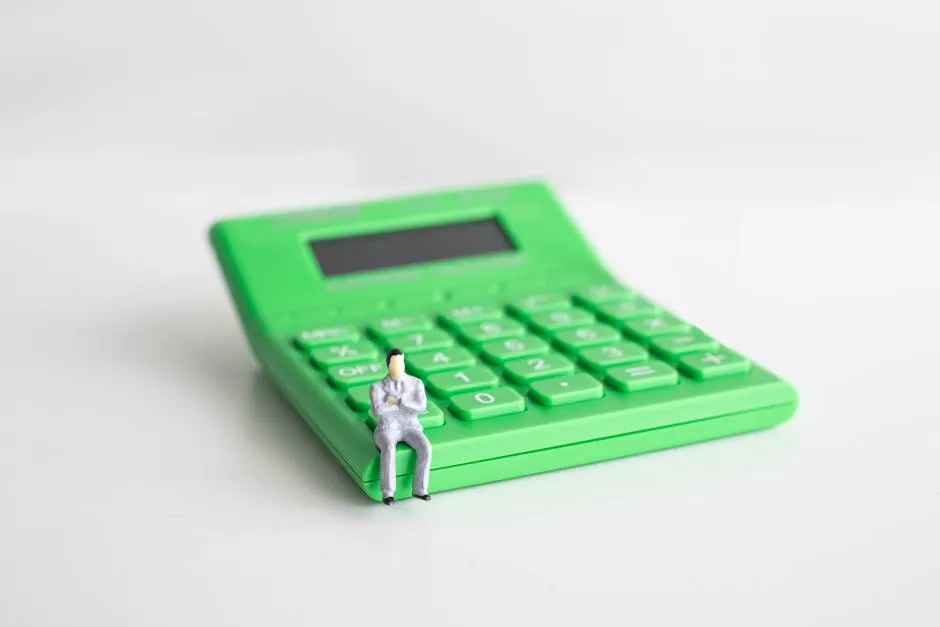
Model evaluation is crucial for understanding model performance. Techniques like cross-validation help assess how well a model generalizes to unseen data. By leveraging these tools, you can build robust statistical models with Python.
For those wanting to dive deeper into machine learning, consider checking out Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow. It’s a fantastic resource for applying machine learning techniques in Python!
Best Practices for Statistical Analysis in Python
Data cleaning and preprocessing are crucial steps in statistical analysis. Raw data often contains errors, duplicates, or missing values. Addressing these issues ensures more accurate results.
Handling missing data is essential. You can use techniques like imputation or deletion. Imputation replaces missing values with mean or median values. Deletion removes rows or columns with missing data. Both methods have pros and cons, so choose wisely.
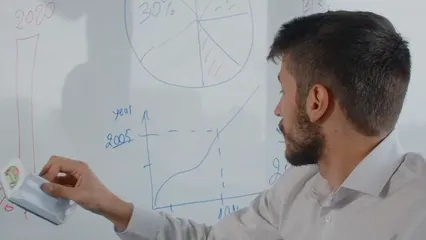
Outliers can skew your analysis. Identifying and managing outliers ensures reliable results. You can use methods like Z-scores or the IQR (Interquartile Range) to detect them. Once identified, decide whether to remove or adjust these values.
To enhance your understanding of data analysis, consider reading The Data Science Handbook: A Guide for Data Scientists. It provides essential insights and practices for data professionals!
Exploratory Data Analysis (EDA) is vital before formal analysis. EDA helps you understand data distributions and relationships. Visualizations and summary statistics are useful during this phase. For a practical example of EDA, refer to the analysis of a sports match:
Check out this analysis of Real Madrid vs Real Sociedad: real madrid vs real sociedad statistics.
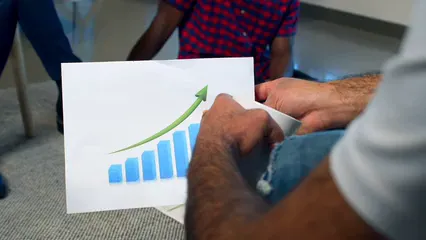
Validating statistical assumptions is important before applying tests. Each statistical test has underlying assumptions, such as normality or homoscedasticity. Ensure your data meets these assumptions to avoid misleading results.
These best practices will enhance your statistical analysis in Python. They lead to more reliable conclusions and informed decision-making.
Conclusion
In this guide, we’ve covered the essentials of Python statistical analysis. We discussed key concepts such as descriptive and inferential statistics. Python’s libraries, like Pandas and SciPy, make these tasks efficient and user-friendly.
Statistical analysis is vital for making informed decisions based on data. It helps businesses identify trends and improve strategies. With Python, you can perform various analyses and gain valuable insights with ease.
We encourage you to explore and practice using Python for statistical analysis. The more you experiment, the more comfortable you’ll become. Dive into projects, harness the power of data, and enhance your skills!
For a comprehensive understanding of data science, don’t miss out on Data Science for Dummies. It’s a great resource for beginners and seasoned pros alike!
FAQs
What is statistical analysis in Python?
Statistical analysis in Python involves techniques to summarize and interpret data. It plays a crucial role in data science. Libraries like Pandas and SciPy are commonly used for these tasks.
How do I perform descriptive statistics in Python?
You can use libraries like Pandas and NumPy. For example, to calculate the mean and standard deviation, you can write: “`python import pandas as pd data = [10, 20, 30, 40, 50] df = pd.Series(data) mean_value = df.mean() std_dev = df.std() “`
What is the difference between descriptive and inferential statistics?
Descriptive statistics summarize data characteristics. Inferential statistics help make predictions or generalizations about a population based on a sample.
Which Python libraries are best for statistical analysis?
Key libraries include: – **NumPy**: For numerical operations. – **Pandas**: For data manipulation. – **SciPy**: For scientific computing. – **StatsModels**: For statistical modeling.
Can Python be used for advanced statistical modeling?
Yes, Python supports advanced techniques like regression analysis and machine learning. Libraries such as StatsModels and Scikit-learn are excellent for these tasks.
How do I visualize statistical data in Python?
You can use libraries like Matplotlib and Seaborn. For example, to create a histogram: “`python import matplotlib.pyplot as plt data = [1, 2, 3, 4, 5] plt.hist(data) plt.show() “`
What are some common pitfalls in statistical analysis?
Common mistakes include ignoring missing data, not validating assumptions, and misinterpreting results. Always check your data and methods to ensure accuracy.
Please let us know what you think about our content by leaving a comment down below!
Thank you for reading till here 🙂
All images from Pexels